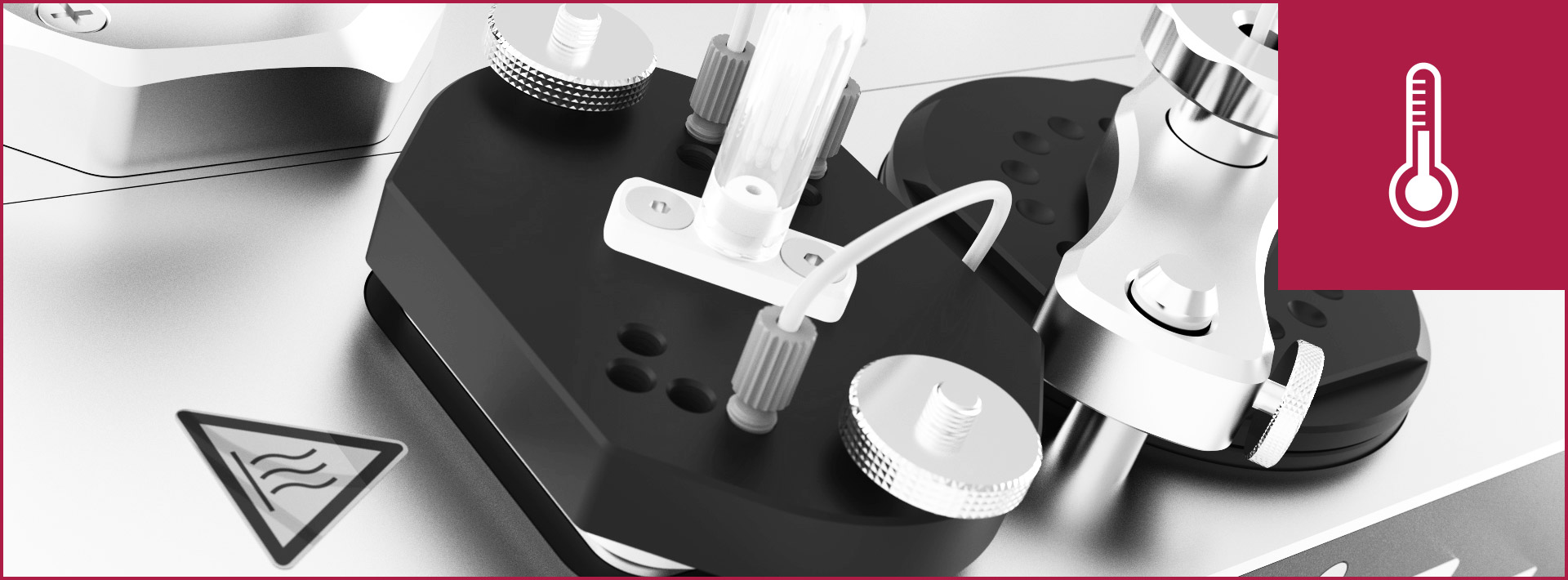
Introduction
This controller library defines a common interface for control device access and configuration.
Including
The way how you include the library functions in your own windows program, depends on the compiler and on the programming language you use. In order to access the controller API, you have to include the library labbCAN_Controller_API.dll to your programming environment. You have to copy this file to the working directory of your system or to the application directory that contains your application EXE file. Use the calling convention LCC_CALL for this library. This convention is managing how the parameters are put on the stack and who is responsible to clean the stack after the function execution.
The interface of the library, constant definitions and declarations of the library functions, is defined in the header file interface/labbCAN_Controller_API.h
Controller API Usage
Retrieve device handle
To work with a controller channel, you need a valid channel handle. A channel handle is simply an opaque pointer to the internal channel object created by the labbCAN environment. So the first thing you need do to is obtaining a valid control channel handle. There are two ways to get a valid channel handle. The first method is to get a channel object via its channel name.
To do this, use the function LCC_LookupChanByName().
You can get the channel names from the device configuration files (see Device Configuration Files). To search for the control channel names, open the file qmixcontroller.xml and search in the channel list for Lcl::CLocalControllerChannel items. The following example shows the device configuration of one single Qmix TC control channel. In the first line you will find the channel name - QmixTC_1_Ctrl1 in this case.
The second method is, to get the channel name by its index. All control channels are number from 0 to n-1 channels. To get a channel by its index, use the function LCC_GetChannelHandle().
If you want to know, how many channels are available, simply call the function LCC_GetNoOfControlChannels(). The following example shows how to use both functions to enumerate all control channels and output their names.
Using Controller Channels
To use the control channel, you simply need to write a setpoint and enable the channel control loop.
Now you can use the function LCC_ReadActualValue() to read the actual control channel value. The following example shows the minimum number of steps to get a control channel running.
Reference
See the labbCAN Controller API module for a detailed reference of all controller control, status and configuration functions.
Dynamically created control channels
The controller library supports the creation of dynamic control channels. A dynamic control channel is a control channel, where the control loop input, control loop output and the controller PID parameters are provided in source code. This enables the dynamic creation of special control loops and thus enables things like pressure controlled dosage with syringe pumps. Dynamic control channels are created with the function LCC_CreatePIDControlChannel(). The following code shows how to create a pressure control loop with an analog input channel that measures the pressure and a syringe pump that generates the flow and the pressure.
To build the control loop you need the device handles for the control loop input and the control loop output. Then you can call the function LCC_CreatePIDControlChannel() to wire input and output together to build up a control loop.
Programming Interface - API
See the labbCAN Controller API module for a detailed reference of the Controller I/O library application programming interface