Creating a device configuration
Before you can use the CETONI SDK you need to create a device configuration. A device configuration is a folder with a set of XML files which contain a complete description of the configuration of all devices in the current setup (see Device Configuration Files for a detailed description).
You can create a valid device configuration with the device configurator of the CETONI Elements software.
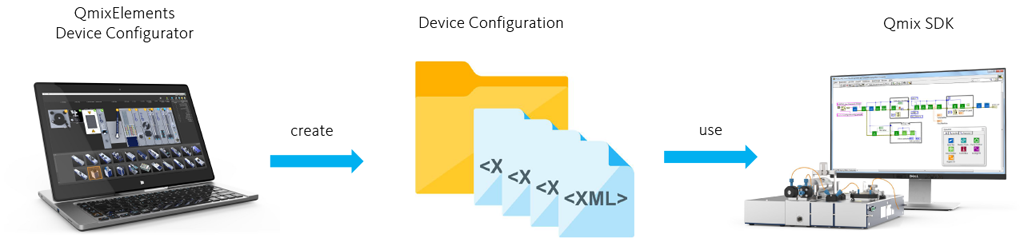
Do the following steps, to create a device configuration
- 1. Start the CETONI Elements software and select Device -> Create Configuration from the main menu.
- 2. Create your device configuration and configure your devices
- 3. Select Device -> Browse Cofiguration Folder from the main menu to find the folder of your new device configuration.
Integration into your development environment
The way how you include the library functions in your own development environment, depends on the compiler and on the programming language you use (see LabVIEW Integration for a detailed description of the CETONI SDK integration into LabVIEW). To access the labbCAN bus API, you have to include the library labbCAN_Bus_API.dll to your programming environment. You have to copy this file to the working directory of your system or to the application directory that contains your application EXE file. Use the calling convention LCB_CALL for this library. This convention is managing how the parameters are put on the stack and who is responsible to clean the stack after the function execution.
The interface of the library, constant definitions and declarations of the library functions, is defined in the header file interface/labbCAN_Bus_API.h
Initialization
To use the DLLs and to access the devices you need to do a certain number of steps to initialize the common bus API and all of the device APIs properly.
- Note
- See labbCAN Bus API for a detailed reference of all labbCAN Bus API specific functions and data structures.
Step 1 - Open the bus library
The first thing you always need to do before you can use any device DLL is to initialize the common labbCAN Bus library by calling the function LCB_Open(). This function
- opens the bus library,
- parses the complete device configuration (a collection of XML files) found in the given configuration directory
- creates all devices and configures them
- establishes the connection to the physical devices
- and sends configuration data to the devices
The following code shows how to open the bus library:
- Note
- The function requires, that you pass in a path to a valid device configuration (see Device Configuration Files). If you do not have a valid device configuration, you need to create one with the CETONI Elements software.
If the function LCB_Open() returns successfully, the labbCAN environment is properly configured and all devices are in pre operational state. In this state the devices are ready for configuration but they do not send any process data.
Step 2 - Retrieve device handles
Now you are ready to setup, configure, and initialize the single devices like single axis systems, analog I/O devices and channels or pumps. You can access each device by a certain "handle". A handle is simply a reference to the internal device object. Therefore the first step for device access is to obtain a device or channel handle. Each device DLL offers a number of functions to obtain a device handle. I.e. the labbCAN Axis System API offers two functions to obtain a valid device handle - LCA_GetAxisSystemHandle() and LCA_LookupAxisSystemByName(). While the first function allows an array like access to all detected positioning systems on the labbCAN bus the second function allows to lookup for a valid device handle by a unique device name.
The following code shows how to obtain an axis system handle by its name:
Normally all devices that are attached to the labbCAN bus are named devices. Each device has a unique device name. If there are a number of devices of the same type the name is suffixed with a number. You can find the names in the XML configuration files (see Device Configuration Files). Each tag <Device>
has an attribute Name
that defines the name of a certain device. The following example snippet from a neMAXYS device configuration file shows the configuration of an axis device with the name neMAXYS1_Y.
See the example labbCAN_CAPI_Init.cpp to learn how to initialize the labbCAN API properly.
Step3 - Setting devices operational
If you have initialized all device APIs and if you finished configuration and setup of all devices then you should finally call the function LCB_Start(). This will start the communication on the bus and all connected devices will start sending their process data periodically or on change of state. After this call, all devices are in state Operational.
Example
The following example code snippet shows a valid initialization procedure:
Shutdown
Just before an applications shuts down it needs to close all labbCAN specific libraries properly to free any claimed resources. To accomplish this an application needs to do the following steps.
Step 1 - Setting devices pre operational
The first step is to call the function LCB_Stop() to stop any further communication. This call will cause all devices to leave Operational state and to go into Pre Operational state.
If you would like to store any device parameters can do this now.
Step 2 - Close bus library
Call the function LCB_Close() to disconnect from device and to free any claimed resources.
- Warning
- Do not access any device specific functions after calling LCB_Close() because the software bus is not connected any longer.
Example
The following example code snippet shows a valid shutdown procedure: