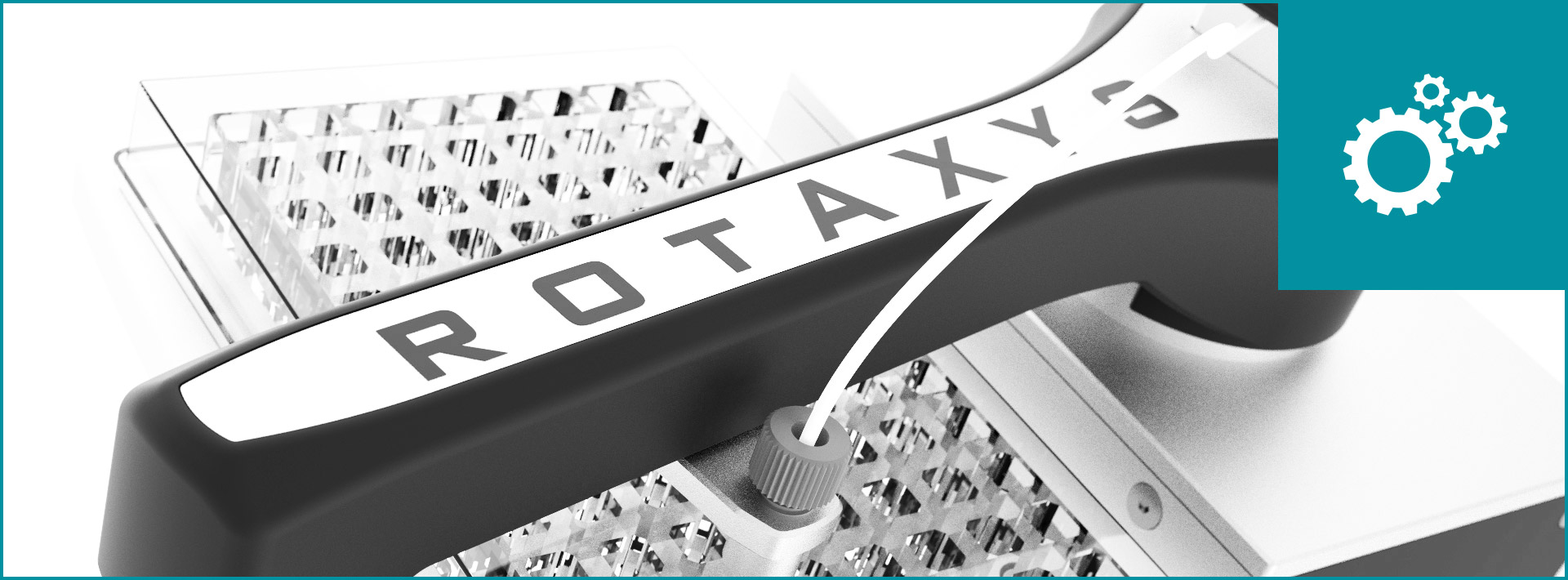
Introduction
The motion control library offers functions and data structures to work with multi axis positioning systems. The API of the motion control library provides functions to work with
- Axis systems (devices that consists of a number of single axes) such as LCA_FindHome() or LCA_MoveToPosXY()
- and single axis devices such as LCA_FindHomeOfAxis() and LCA_MoveToPos()
So depending on the requirements of your application, you can work with the single axis interface or with the multi axis interface. For some devices such as the rotAXYS axis system it is much easier to use the multi axis interface for positioning in XY space. Because the rotAXYS axis system has a rotation axis, some translations needs to take place to convert between polar and Cartesian coordinates. If you work with the single axis devices, you need to do these translations for yourself. If you work with the multi axis interface (LCA_MoveToPosXY()), then the axis system handles all the translation stuff internally.
- See also
- See Devices page for detailed informations about specific motion control devices.
Including
The way how you include the library functions in your own windows program, depends on the compiler and on the programming language you use. In order to access the motion control API, you have to include the library labbCAN_MotionControl_API.dll to your programming environment. You have to copy this file to the working directory of your system or to the application directory that contains your application EXE file. Use the calling convention LCA_CALL for this library. This convention is managing how the parameters are put on the stack and who is responsible to clean the stack after the function execution.
The interface of the library, constant definitions and declarations of the library functions, is defined in the header file interface/labbCAN_MotionControl_API.h
Initialization
Step 1 - Retrieve device handle
To work with axis systems or single axis devices, you need valid device handles. A device handle is simply an opaque pointer to the internal device object created by the labbCAN environment. So the first thing you need do to is obtaining a valid device handle.
You can get a valid axis system handle with the functions LCA_LookupAxisSystemByName() or LCA_GetAxisSystemHandle():
You can get a valid axis handle, if you obtain a certain axis from a given axis system device and a given axis index:
or if you query the global object pool for a registered named axis object:
- Note
- You can get the axis names and axis system names from the device configuration files (see Device Configuration Files) that have been shipped with your devices.
Step 2 - Enable devices
If you have valid device handles, you can start to bring your devices into a properly enabled and initialized state. To do this, you need to:
- clear all faults (e.g. LCA_ClearAxisFault())
- enable all drives (LCA_Enable())
- execute the homing procedure to find the device homing positions (LCA_FindHome())
If you work with the multi axis functions, you simply need to call LCA_FindHome(). This function will
- clear the axis faults of all devices that belong to the given axis system
- enable all axes of the axis system
- execute the homing procedure of the given axis system.
After the homing procedure the device is properly initialized an you can start with your normal motion control tasks.
Examples
The following example code snippet shows a valid axis system initialization procedure:
Reference
See the Axis System Initialization module for a detailed reference of all motion control functions for initialization.
Programming Interface - API
See the labbCAN Motion Control API module for a detailed reference of the motion control library application programming interface